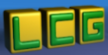 |
Java
1.0
|
Go to the documentation of this file.
4 import java.util.IllegalFormatException;
5 import java.util.regex.Matcher;
6 import java.util.regex.Pattern;
8 import javax.swing.JFileChooser;
9 import javax.swing.JOptionPane;
45 public final static char EOF = (char)0xFFFF;
51 public final static char EOLN =
'\n';
81 if (inputStream ==
null)
84 readStream(
new InputStreamReader(inputStream));
94 if (inputStream ==
null)
97 if ( inputStream instanceof BufferedReader)
98 in = (BufferedReader)inputStream;
100 in =
new BufferedReader(inputStream);
118 if (fileName ==
null)
121 BufferedReader newin;
123 newin =
new BufferedReader(
new FileReader(fileName) );
125 catch (Exception e) {
126 throw new IllegalArgumentException(
"Can't open file \"" + fileName +
"\" for input.\n"
127 +
"(Error :" + e +
")");
133 catch (Exception e) {
162 fileDialog.setDialogTitle(
"Select File for Input");
164 if (option != JFileChooser.APPROVE_OPTION)
166 File selectedFile =
fileDialog.getSelectedFile();
167 BufferedReader newin;
169 newin =
new BufferedReader(
new FileReader(selectedFile) );
171 catch (Exception e) {
172 throw new IllegalArgumentException(
"Can't open file \"" + selectedFile.getName() +
"\" for input.\n"
173 +
"(Error :" + e +
")");
179 catch (Exception e) {
201 catch (Exception e) {
217 if (outputStream ==
null)
230 if (outputStream ==
null)
255 if (fileName ==
null)
260 newout =
new PrintWriter(
new FileWriter(fileName));
262 catch (Exception e) {
263 throw new IllegalArgumentException(
"Can't open file \"" + fileName +
"\" for output.\n"
264 +
"(Error :" + e +
")");
270 catch (Exception e) {
294 fileDialog.setDialogTitle(
"Select File for Output");
298 if (option != JFileChooser.APPROVE_OPTION)
301 if (selectedFile.exists()) {
302 int response = JOptionPane.showConfirmDialog(
null,
303 "The file \"" + selectedFile.getName() +
"\" already exists. Do you want to replace it?",
304 "Replace existing file?",
305 JOptionPane.YES_NO_OPTION, JOptionPane.WARNING_MESSAGE);
306 if (response == JOptionPane.YES_OPTION)
315 newout =
new PrintWriter(
new FileWriter(selectedFile));
317 catch (Exception e) {
318 throw new IllegalArgumentException(
"Can't open file \"" + selectedFile.getName() +
"\" for output.\n"
319 +
"(Error :" + e +
")");
325 catch (Exception e) {
361 public static void put(Object x) {
364 if (
out.checkError())
379 public static void put(Object x,
int minChars) {
383 out.printf(
"%" + minChars +
"s", x);
385 if (
out.checkError())
392 public static void putln(Object x) {
395 if (
out.checkError())
402 public static void putln(Object x,
int minChars) {
406 if (
out.checkError())
416 if (
out.checkError())
429 public static void putf(String format, Object... items) {
431 throw new IllegalArgumentException(
"Null format string in TextIO.putf() method.");
433 out.printf(format,items);
435 catch (IllegalFormatException e) {
436 throw new IllegalArgumentException(
"Illegal format string in TextIO.putf() method.");
439 if (
out.checkError())
451 return peek() ==
'\n';
459 public static boolean eof() {
494 while (ch !=
EOF && ch !=
'\n' && Character.isWhitespace(ch)) {
508 while (ch !=
EOF && Character.isWhitespace(ch)) {
646 StringBuffer s =
new StringBuffer(100);
682 return (
int)
readInteger(Integer.MIN_VALUE, Integer.MAX_VALUE);
692 return readInteger(Long.MIN_VALUE, Long.MAX_VALUE);
718 "Real number in the range " + (-Float.MAX_VALUE) +
" to " + Float.MAX_VALUE);
722 x = Float.parseFloat(str);
724 catch (NumberFormatException e) {
725 errorMessage(
"Illegal floating point input, " + str +
".",
726 "Real number in the range " + (-Float.MAX_VALUE) +
" to " + Float.MAX_VALUE);
729 if (Float.isInfinite(x)) {
730 errorMessage(
"Floating point input outside of legal range, " + str +
".",
731 "Real number in the range " + (-Float.MAX_VALUE) +
" to " + Float.MAX_VALUE);
753 "Real number in the range " + (-Double.MAX_VALUE) +
" to " + Double.MAX_VALUE);
757 x = Double.parseDouble(str);
759 catch (NumberFormatException e) {
760 errorMessage(
"Illegal floating point input, " + str +
".",
761 "Real number in the range " + (-Double.MAX_VALUE) +
" to " + Double.MAX_VALUE);
764 if (Double.isInfinite(x)) {
765 errorMessage(
"Floating point input outside of legal range, " + str +
".",
766 "Real number in the range " + (-Double.MAX_VALUE) +
" to " + Double.MAX_VALUE);
785 StringBuffer str =
new StringBuffer(50);
787 while (ch ==
EOF || !Character.isWhitespace(ch)) {
791 return str.toString();
807 if ( s.equalsIgnoreCase(
"true") || s.equalsIgnoreCase(
"t") ||
808 s.equalsIgnoreCase(
"yes") || s.equalsIgnoreCase(
"y") ||
813 else if ( s.equalsIgnoreCase(
"false") || s.equalsIgnoreCase(
"f") ||
814 s.equalsIgnoreCase(
"no") || s.equalsIgnoreCase(
"n") ||
821 "one of: true, false, t, f, yes, no, y, n, 0, or 1");
834 private final static BufferedReader
standardInput =
new BufferedReader(
new InputStreamReader(System.in));
848 private final static Pattern
integerRegex = Pattern.compile(
"(\\+|-)?[0-9]+");
849 private final static Pattern
floatRegex = Pattern.compile(
"(\\+|-)?(([0-9]+(\\.[0-9]*)?)|(\\.[0-9]+))((e|E)(\\+|-)?[0-9]+)?");
852 private static int pos = 0;
892 "Integer in the range " + min +
" to " + max);
895 String str = s.toString();
897 x = Long.parseLong(str);
899 catch (NumberFormatException e) {
901 "Integer in the range " + min +
" to " + max);
904 if (x < min || x > max) {
905 errorMessage(
"Integer input outside of legal range, " + str +
".",
906 "Integer in the range " + min +
" to " + max);
921 out.print(
" *** Error in input: " + message +
"\n");
922 out.print(
" *** Expecting: " + expecting +
"\n");
923 out.print(
" *** Discarding Input: ");
925 out.print(
"(end-of-line)\n\n");
931 out.print(
"Please re-enter: ");
936 throw new IllegalArgumentException(
"Too many input consecutive input errors on standard input.");
939 throw new IllegalArgumentException(
"Error while reading from file \"" +
inputFileName +
"\":\n"
940 + message +
"\nExpecting " + expecting);
942 throw new IllegalArgumentException(
"Error while reading from inptu stream:\n"
943 + message +
"\nExpecting " + expecting);
961 throw new IllegalArgumentException(
"Attempt to read past end-of-file in standard input???");
963 throw new IllegalArgumentException(
"Attempt to read past end-of-file in file \"" +
inputFileName +
"\".");
973 catch (Exception e) {
975 throw new IllegalArgumentException(
"Error while reading standard input???");
977 throw new IllegalArgumentException(
"Error while attempting to read from file \"" +
inputFileName +
"\".");
979 throw new IllegalArgumentException(
"Errow while attempting to read form an input stream.");
992 System.err.println(
"Error occurred in TextIO while writing to standard output!!");
996 throw new IllegalArgumentException(
"Too many errors while writing to standard output.");
1000 throw new IllegalArgumentException(
"Error occurred while writing to file \""
1004 throw new IllegalArgumentException(
"Error occurred while writing to output stream:\n " + message);
static void writeStandardOutput()
After this method is called, output will be written to standard output (as it is in the default state...
static char getChar()
Skips whitespace characters and then reads a single non-whitespace character from input.
static Matcher integerMatcher
static long getLong()
Skips whitespace characters and then reads a value of type long from input.
static short getlnShort()
Skips whitespace characters and then reads a value of type short from input, discarding the rest of t...
static String inputFileName
static Matcher floatMatcher
static void writeStream(PrintWriter outputStream)
After this method is called, output will be sent to outputStream, provided it is non-null.
static boolean writeUserSelectedFile()
Puts a GUI file-selection dialog box on the screen in which the user can select an output file.
static final char EOLN
The value returned by the peek() method when the input is at end-of-line.
static String getlnString()
This is identical to getln().
static boolean getlnBoolean()
Skips whitespace characters and then reads a value of type boolean from input, discarding the rest of...
static long readInteger(long min, long max)
static String getOutputFileName()
If TextIO is currently writing to a file, then the return value is the name of the file.
static void readFile(String fileName)
Opens a file with a specified name for input.
static boolean eof()
Test whether the next character in the current input source is an end-of-file.
static void putf(String format, Object... items)
Writes formatted output values to the current output destination.
static void writeFile(String fileName)
Opens a file with a specified name for output.
static String outputFileName
static void put(Object x)
Write a single value to the current output destination, using the default format and no extra spaces.
static void putln()
Write an end-of-line character to the current output destination.
static void put(Object x, int minChars)
Write a single value to the current output destination, using the default format and outputting at le...
static void writeStream(OutputStream outputStream)
After this method is called, output will be sent to outputStream, provided it is non-null.
static String readRealString()
static boolean readUserSelectedFile()
Puts a GUI file-selection dialog box on the screen in which the user can select an input file.
static String getInputFileName()
If TextIO is currently reading from a file, then the return value is the name of the file.
static float getFloat()
Skips whitespace characters and then reads a value of type float from input.
static double getDouble()
Skips whitespace characters and then reads a value of type double from input.
static void outputError(String message)
static JFileChooser fileDialog
static boolean writingStandardOutput
static void skipBlanks()
Skips over any whitespace characters, except for end-of-lines.
static byte getlnByte()
Skips whitespace characters and then reads a value of type byte from input, discarding the rest of th...
TextIO provides a set of static methods for reading and writing text.
static void readStandardInput()
After this method is called, input will be read from standard input (as it is in the default state).
static void errorMessage(String message, String expecting)
static void skipWhitespace()
Skips over any whitespace characters, including for end-of-lines.
static boolean readingStandardInput
static void emptyBuffer()
static void putln(Object x)
This is equivalent to put(x), followed by an end-of-line.
static long getlnLong()
Skips whitespace characters and then reads a value of type long from input, discarding the rest of th...
static boolean getBoolean()
Skips whitespace characters and then reads a value of type boolean from input.
static byte getByte()
Skips whitespace characters and then reads a value of type byte from input.
static int inputErrorCount
static float getlnFloat()
Skips whitespace characters and then reads a value of type float from input, discarding the rest of t...
static short getShort()
Skips whitespace characters and then reads a value of type short from input.
static String getWord()
Skips whitespace characters and then reads one "word" from input.
static int outputErrorCount
static double getlnDouble()
Skips whitespace characters and then reads a value of type double from input, discarding the rest of ...
static char getlnChar()
Skips whitespace characters and then reads a value of type char from input, discarding the rest of th...
static char peek()
Returns the next character in the current input source, without actually removing that character from...
static void readStream(Reader inputStream)
After this method is called, input will be read from inputStream, provided it is non-null.
static final PrintWriter standardOutput
static void putln(Object x, int minChars)
This is equivalent to put(x,minChars), followed by an end-of-line.
static String getlnWord()
Skips whitespace characters and then reads one "word" from input, discarding the rest of the current ...
static final char EOF
The value returned by the peek() method when the input is at end-of-file.
static int getlnInt()
Skips whitespace characters and then reads a value of type int from input, discarding the rest of the...
static final Pattern floatRegex
static final BufferedReader standardInput
static String readIntegerString()
static char getAnyChar()
Reads the next character from the current input source.
static final Pattern integerRegex
static void readStream(InputStream inputStream)
After this method is called, input will be read from inputStream, provided it is non-null.
static int getInt()
Skips whitespace characters and then reads a value of type int from input.
static boolean eoln()
Test whether the next character in the current input source is an end-of-line.
static String getln()
Reads all the characters from the current input source, up to the next end-of-line.